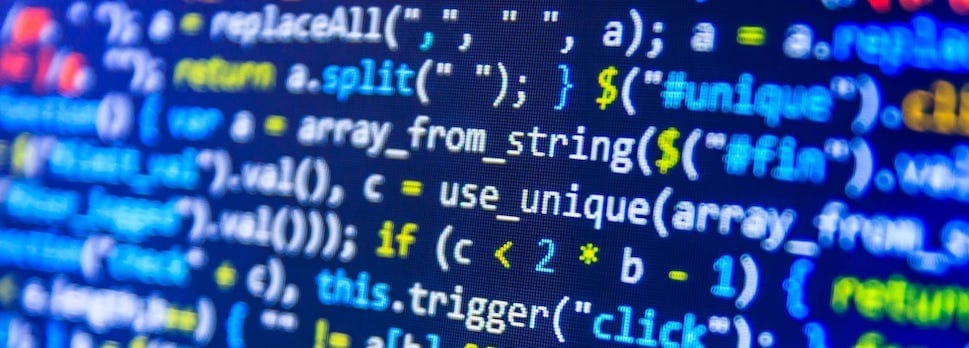
Long methods vs simple ones. What’s the problem?
Long methods’ issues:
- usually does too much and “knows” too much
- as a consequence, it breaks the Single Responsibility Principle
- it is harder to grasp what it does when you read it
- more changes needed to add or amend functionality
- you have to write pretty complex tests
- and you need a lot of them to cover all of the possible permutations
# RuntimeError < StandardError < Exception < Object
begin
require 'does/not/exist'
rescue Exception => e
"it is an Exception"
end
# => "it is an Exception"
begin
require 'does/not/exist'
rescue StandardError => e
"it is an Exception"
end
# => LoadError: cannot load such file -- does/not/exist
What is the difference in results between this
and this?
require 'does/not/exist' rescue "Hi"
And why the results are different. Let’s figure it out step by step.
a = Stack() # []
a.push('one') # ['one']
a.push('two') # ['one', 'two']
a.top() # 'two'
a.push('three') # ['one', 'two', 'three']
a.top() # 'three'
a.size() # 3
a.pop() # 'three' ['one', 'two']
a.pop() # 'two' ['one']
This is the API I want to have for the ‘Stack’ data structure.
Let’s implement it in Python
step by step.
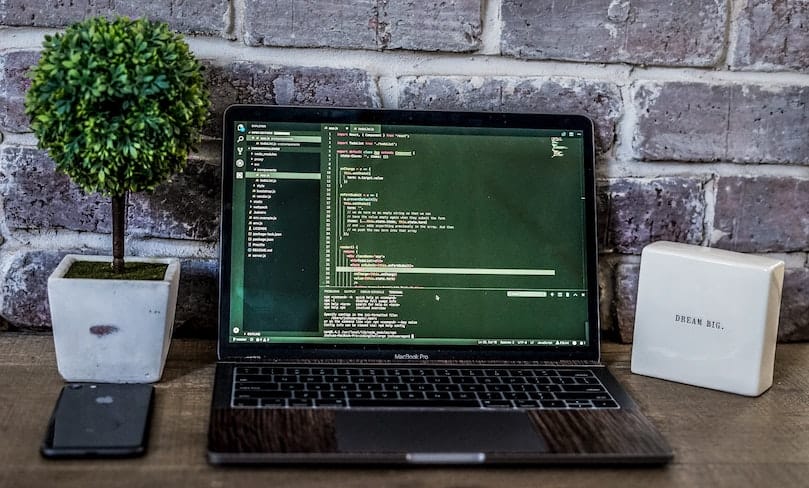
In the previous JavaScript like object in Ruby on Rails article,
I’ve described how similar behavior can be achieved in Rails code.
But in pure Ruby there still is no short way for this.
It turns out that a proposal to Ruby core has been made to add this nice-to-have feature.
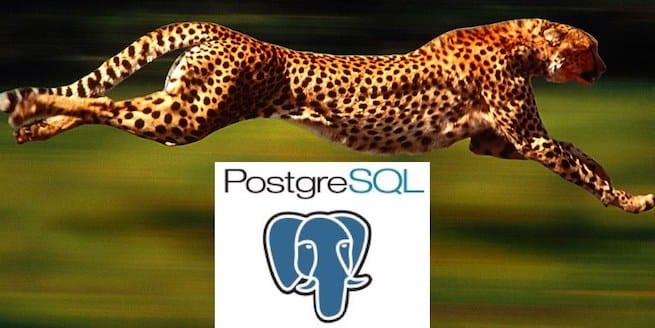
Today I’ve learned about one of the useful PostgreSQL features - ‘unlogged’ tables.
What does this feature exist for?
Say you need to cache some transient data and you don’t really care about it being persisted after a server crash,
enter ‘unlogged’ tables.
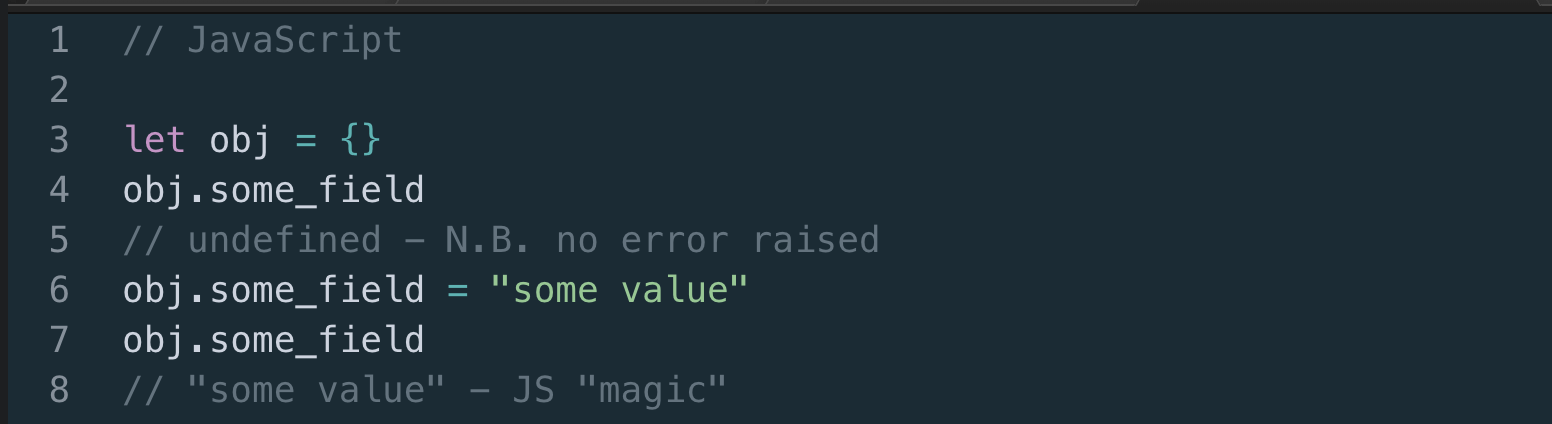
One day, when I was explaining how the routing > params > controllers > views
work,
I wanted to keep things as simple as possible,
so I needed a way to flexibly “mock” AR objects.
And the JavaScript-like objects would be a good-enough solution for it.
let obj = {}
obj.some_field
// undefined - N.B. no error raised
obj.some_field = "some value"
obj.some_field
// "some value" - JS "magic"
In contrast, the Ruby’s Object does not allow it.

I was explaining what the rails db:migrate
command does under the hood
and I wanted to demonstrate what SQL queries the Rails generates.
And it would be nice for them to be colorful of course 😃
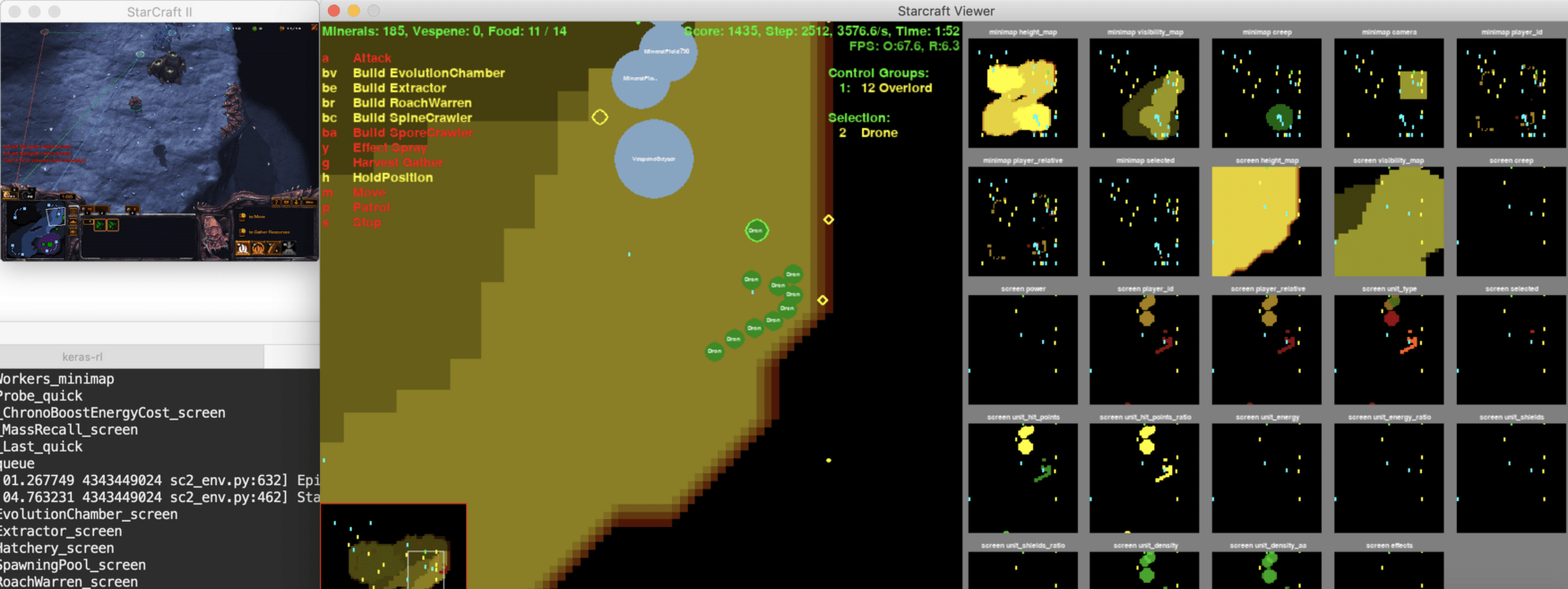
This a bit of pretentious title is about the setup of the great PySC2 library
which makes it possible to “rule” the StarCraft II with your own AI.
“Scientifically” speaking, the PySC2 allows for Reinforcement Learning agents
to interact with the StarCraft II
game.
The library does it by exposing the StarCraft II Machine Learning API
as a Python RL Environment.
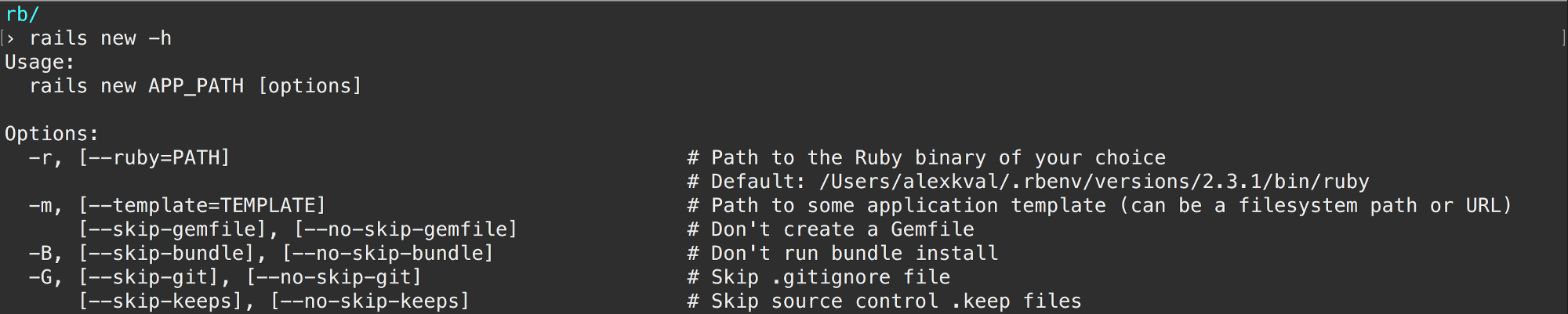
If we run rails new -h
we see that we can disable generating of some parts of a new rails project.
In this article I am going to dissect these particular options:
-S, [--skip-sprockets] # Skip Sprockets files
-J, [--skip-javascript] # Skip JavaScript files
[--skip-turbolinks] # Skip turbolinks gem
-O, [--skip-active-record] # Skip Active Record files